一、 JSX和组件的概念
React的核心机制就是虚拟DOM,可以在内存中创建的虚拟DOM元素,React利用虚拟DOM来减少对实际DOM的操作从而提升性能。传统的创建方式是:
var newStar = document.createElement('div');
newStar.className = 'star';
document.getElementById('main').appendChild(newStar);
var pic = document.createElement('img');
img.src="https://www.google.com/images/branding/googlelogo/2x/googlelogo_color_272x92dp.png";
img.className='avatar';
document.getElementById('main').appendChild(pic);
但是这样代码的可读性并不是很理想,于是React发明了JSX,利用我们熟悉的HTML语法来创建虚拟DOM:
var root = (
<div id="main">
<div className="star">
</div>
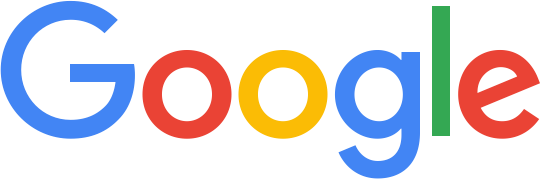
</div>
)
在实际开发中,JSX在产品打包阶段都已编译成纯Javascript,JSX的语法不会带来任何的性能影响。
二。 View组件中常见的属性。
React Native中的组件View,它相当于iOS中的UIView,android中的Android.View,html中的div标签,它是所有组件的父组件。
Flexbox 弹性布局
ShadowProp (支持ios)阴影
Transform 动画属性
backfaceVisibility enum('visible', 'hidden')
backgroundColor string
borderColor string
borderTopColor string
borderRightColor string
borderBottomColor string
borderLeftColor string
borderRadius number
borderTopLeftRadius number
borderTopRightRadius number
borderBottomLeftRadius number
borderBottomRightRadius number
borderStyle enum('solid', 'dotted', 'dashed')
borderWidth number
borderTopWidth number
borderRightWidth number
borderBottomWidth number
borderLeftWidth number
opacity number
overflow enum('visible', 'hidden')
等:具体属性详见 [React Native 中文网](http://reactnative.cn/docs/0.46/view.html#content)
三、View组件的运用
export default class RNTestDemo extends Component {
render() {
return (
<View style={{flex:1,backgroundColor:'green',padding:20}}>
<View style={{width:300,height:50,backgroundColor:"blue"}}></View>
<View style={{width:100,height:50,backgroundColor:"yellow",marginTop:10}}></View>
</View>
);
}
}
在代码的render函数中,我们返回了一个顶层(原谅色)的View,然后这个父视图中包含了另外两个(蓝色和黄色的)两个子View。
在顶层View中的style属性里面设置了占满父控件的属性(flex:1), 内边距为20,背景颜色为原谅色。对应子层中的蓝色视图,宽为300,高度50。�子层中的黄色视图,上边距为10。
下图是运行效果:
屏幕快照 2017-07-17 上午11.36.22.png
在React native中,采用StyleSheet来进行布局能使我们的代码更加规范和利于维护,如:
/**
* Sample React Native App
* https://github.com/facebook/react-native
* @flow
*/
import React, { Component } from 'react';
import {
AppRegistry,
StyleSheet,
Text,
View
} from 'react-native';
export default class RNTestDemo extends Component {
render() {
return (
<View style={styles.container}>
<View style={styles.blueViewStyle}></View>
<View style={styles.yellowViewStyle}></View>
</View>
);
}
}
const styles = StyleSheet.create({
container:{
flex:1,
backgroundColor:'green',
padding:20
},
blueViewStyle:{
width:300,
height:50,
backgroundColor:"blue"
},
yellowViewStyle:{
width:100,
height:50,
backgroundColor:"yellow",
marginTop:10
}
});
AppRegistry.registerComponent('RNTestDemo', () => RNTestDemo);